Enhancement/issue 122 filter for requests #133
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
Hello
I have created filter UI and filter logic for the responses as requested in Issue 122.
I tried to take a dynamic approach without affecting existing logic and models; filter models are created by predetermined categories (code and method in current version) and from observed request model.
I am looking forward to your feedback.
UI
Simulator.Screen.Recording.-.iPhone.14.Pro.-.2023-03-12.at.12.58.23.mp4
I did use popover view that anchored to filter button on SearchBar. View height is dynamic and consists of 2 level:
You can access from this Figma link for the design.
Level 1 Categories
Level 2 Types (Values)
Logic
You can access this logic flow with this Figma link
Model
Filters are represented with FilterModel.
On ViewModel array of FilterModel gets stored in the FilterCollectionModel.
Flow
A new FilterModel gets created with a didSet observer for Requests. Created array of filterModel gets saved or updated then filterChange notification gets posted.
On ViewController, filterChange notification being observed. On post, FilterCollectionModel gets created from filter data and existing request data gets updated via filter data and search text.
When user selects a filter type from FilterTypeViewController, cell UI gets updated on didSelectRowAt and after animation update gets requestested for selected filter, filter selectionStatus gets updated with "updateFilterModel" method.
How to add new category and types for category?
While explaining the logic, I will give example with scheme parameter of the requests.
Category
Category is Enum type with String desc for each case. We have to add new case to Enum and case array on FilterViewController.
FilterModel.swift
FilterViewController.swift
Final result for adding category:
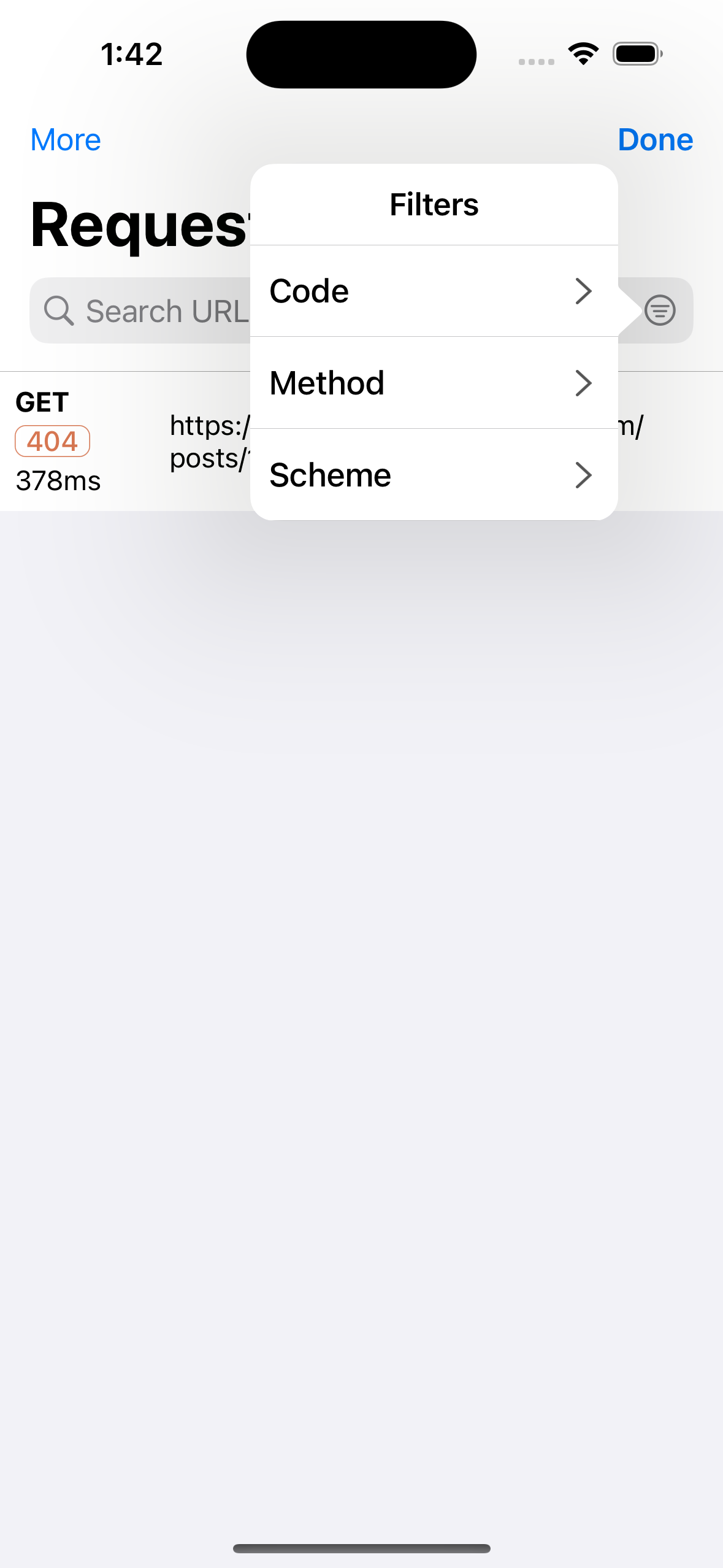
Category Types
For category types saving, updating and UI methods are handled dynamically, but we have add conditions and Equatable types for filter model creation and data filtering methods.
Final result of "createFilterModel" method:
We can see the scheme types in FilterTypeViewController but selecting type doesn't affect the logic yet.
To effect the logic we have to:
Final result of FilterCollectionModel:
new we can add scheme conditions to filterByFilterModels on RequestsViewController handle filtering of the data.
Final result of "filterByFilterModels"
P.S. I have added some lightweight extensions to library classes to ease the development of the UI part.