-
Notifications
You must be signed in to change notification settings - Fork 252
Visual Studio Code
Install the ccls extension from the marketplace here. The source code is located at https://github.com/MaskRay/vscode-ccls.
If you run into issues, you can view debug output by running the
(F1
) View: Toggle Output
command and opening the ccls
output section.
To use the vscode-ccls extension without installing it, you can launch VS code from the command-line with the path to local copy of vscode-ccls repository as a command argument. Example:
code vscode-ccls
Then press F5 to launch a copy of vscode with the plugin temporarily installed. To use vscode-ccls, you can open your project in the new instance of vscode.
You probably will want to configure the vscode-ccls plugin before initial use. To access the configuration while running VS Code, this may be performed via the Command Pallete. (Hint: "Ctrl+Shift+P", or View->Command Pallete. Update: with the current vscode version: "Ctrl+,", or File->Preferences->Settings), and enter the following text:
@ext:ccls-project.ccls
After changing some ccls configurations in settings.json
, trigger the command ccls: Restart language server
or reload the plugin to take effect.
To tell the extension where to find ccls, either add ccls to your PATH
or set "ccls.launch.command" in User Settings to the absolute path pointing to ccls.
"ccls.launch.command": "/path/to/ccls/Release/ccls",
By default, ccls will store indexes in .ccls-cache
of the workspace (more technically, the current working directory of ccls, which may not be what you think is the workspace root).
You can set:
"ccls.cache.directory": "${workspaceFolder}/.ccls-cache/"
or use a global cache directory:
// Linux, *BSD
"ccls.cache.directory": "${env:HOME}/.cache/ccls-cache",
// macOS
"ccls.cache.directory": "${env:TMPDIR}/.cache/ccls-cache",
// Windows
"ccls.cache.directory": "${env:TEMP}/ccls-cache",
If for whatever reason you cannot generate a compile_commands.json
file, you
can add the flags to the ccls.clang.extraArgs
configuration
option.
In case compile_commands.json
is located not in default place ($root)
"ccls.misc.compilationDatabaseDirectory": "build"
In this case it is located in $root/build/compile_commands.json
Now you may start using the features of vscode-ccls with your development project.
- Open the project root with "Open Folder" (C-k C-o)
On Macos you might run into issues with certain includes (stddef.h stdint.h
) not being found. To fix this you need to set the resource directory to the output of clang -print-resource-dir
. The default value is hardcoded at ccls compile time, so unless you compile it yourself this will not work.
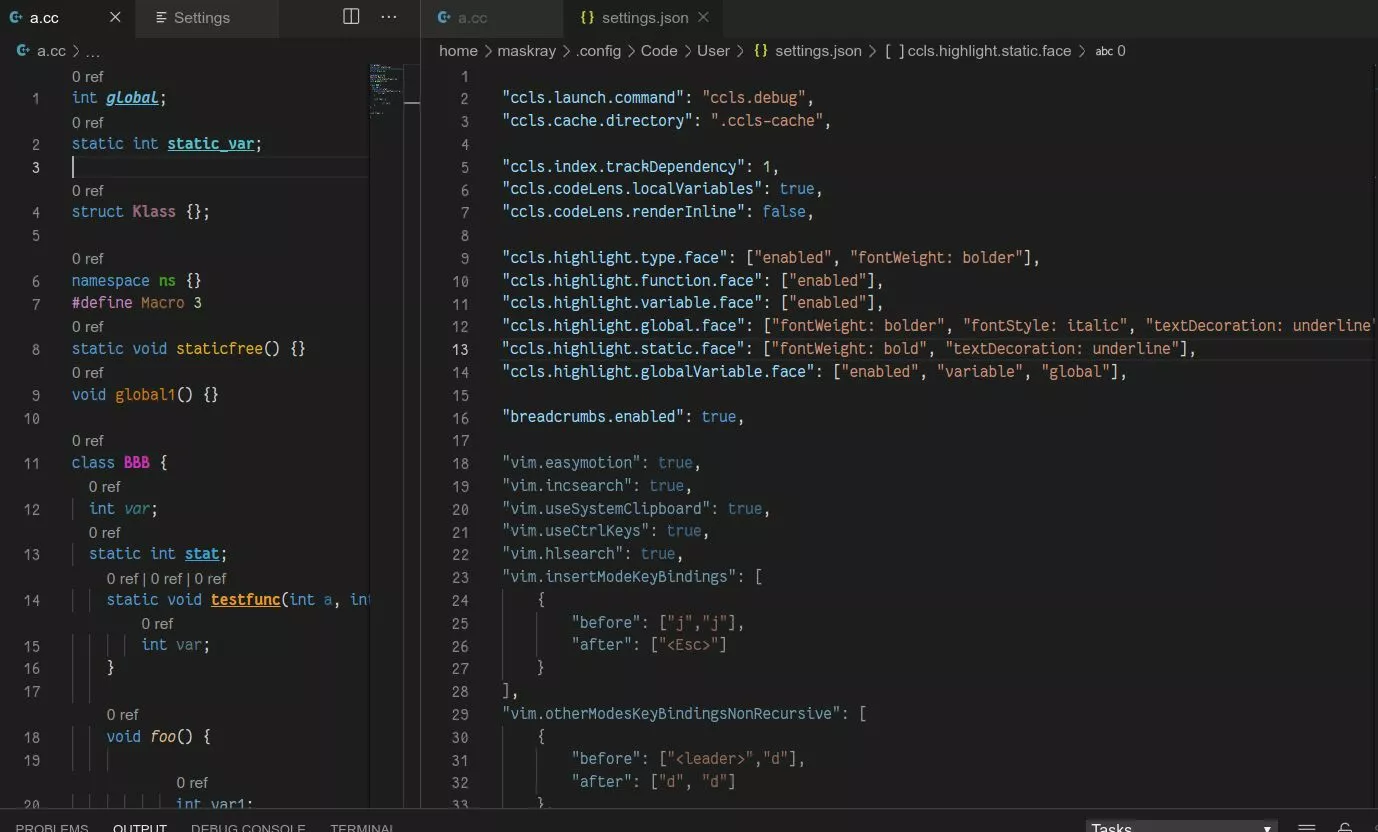
Add the following lines to settings.json
to enable rainbow semantic highlight:
"ccls.highlight.function.face": ["enabled"],
"ccls.highlight.type.face": ["enabled"],
"ccls.highlight.variable.face": ["enabled"],
Before vscode-ccls 0.1.28, settings like "ccls.highlighting.enabled.types": true,
were used.
ccls.highlight.{function,type,type}.face
define faces of basic symbol kinds
from which other symbol kinds ({member,staticMember}{Function,Variable}
, etc)
can inherit. package.json
defines some default faces:
// Predefined by package.json
"ccls.highlight.member": ["fontStyle: italic"],
"ccls.highlight.static": ["fontWeight: bold"],
"ccls.highlight.memberFunction.face": ["function", "member"]
"ccls.highlight.memberVariable.face": ["variable", "member"]
"ccls.highlight.staticMemberFunction.face": ["function", "static"]
"ccls.highlight.staticMemberVariable.face": ["variable", "static"]
For a symbol kind, its face property takes effect if "enabled"
is specified either directly or indirectly. The face describes:
- Styles such as
"fontStyle: italic"
,"fontWeight: bolder"
, and"textDecoration: underline"
. They are based on CSS properties with similar names. See MDN documents about font-weight and text-decoration. - Colors. By default,
package.json
predefines some palettes.ccls.highlight.memberFunction.colors
is not defined but it can inherit fromccls.highlight.function.colors
becauseccls.highlight.memberFunction.face
inherits fromccls.highlight.function.face
.
// Predefined by package.json
"ccls.highlight.function.colors": ["#e5b124", "#927754", "#eb992c", "#e2bf8f", "#d67c17", "#88651e", "#e4b953", "#a36526", "#b28927", "#d69855"],
"ccls.highlight.type.colors": ["#e1afc3", "#d533bb", "#9b677f", "#e350b6", "#a04360", "#dd82bc", "#de3864", "#ad3f87", "#dd7a90", "#e0438a"],
"ccls.highlight.variable.colors": ["#587d87", "#26cdca", "#397797", "#57c2cc", "#306b72", "#6cbcdf", "#368896", "#3ea0d2", "#48a5af", "#7ca6b7"],
"ccls.highlight.macro.colors": ["#e79528", "#c5373d", "#e8a272", "#d84f2b", "#a67245", "#e27a33", "#9b4a31", "#b66a1e", "#e27a71", "#cf6d49"],
"ccls.highlight.namespace.colors": ["#429921", "#58c1a4", "#5ec648", "#36815b", "#83c65d", "#417b2f", "#43cc71", "#7eb769", "#58bf89", "#3e9f4a"],
The colors will be randomly assigned to symbols. Change these properties to 1-element arrays to make colors fixed.
The list of available symbol kinds: function, variable, type, enum, globalVariable, macro, memberFunction, memberVariable, namespace, parameter, staticMemberFunction, staticVariable, typeAlias
.
globalVariable
works with latest ccls but not the 0.20190823.3 release.
You could set custom lookup using keybindings. Use command palette (ctrl + shift + p
by default) -> Open keyboard shortcuts file
// functions called by current selected function, up to 3rd level.
{"key":"<ctrl-shift-alt-c>","command":"ccls.call","args":{"callee":true,"levels":3}}
// functions that call this function. This is what "Show Cross References" shows by default
{"key":"<your-shortcuts>","command":"ccls.call"}
// see inheritance instead of base
{"key":"<your-shortcuts>","command":"ccls.base","args":{"derived":true,"levels":2}}
// nested classes / types in a namespace (kind: 2)
{"key":"<your-shortcuts>","command":"ccls.member","args":{"kind":2}}
// member functions / functions in a namespace(kind 3)
{"key":"<your-shortcuts>","command":"ccls.member","args":{"kind":3}}
For VSCodeVim users, heres how to set arguments in User Settings (settings.json
)
"vim.normalModeKeyBindingsNonRecursive": [
{
"before":["<leader>","t"],
"commands":[{"command":"ccls.call","args":{"levels":2,"callee":true}}]
}
]
So you could hit <space>-t
to see callees up to 3rd level if you set <leader>
to <space>
The vscode-ccls
extension doesnt provide any debugger integration. One popular option is to use the vscode-cpptools extension for this aspect. By default however, vscode-cpptools
provides editor features that overlap with ccls, which yields duplicate results in things like autocomplete. The following settings may be applied to disable the editor support of vscode-cpptools
to prevent this from happening, while still retaining the debugger features.
"C_Cpp.autocomplete": "Disabled",
"C_Cpp.formatting": "Disabled",
"C_Cpp.errorSquiggles": "Disabled",
"C_Cpp.intelliSenseEngine": "Disabled",
The command is ccls.navigate
, which need an argument direction
. ("D" => first child declaration "L" => previous declaration "R" => next declaration "U" => parent declaration)
"vim.normalModeKeyBindingsNonRecursive": [
{"before":["<leader>","j"],"commands":[{"command":"ccls.navigate","args":{"direction":"R"}}]},
{"before":["<leader>","k"],"commands":[{"command":"ccls.navigate","args":{"direction":"L"}}]},
{"before":["<leader>","h"],"commands":[{"command":"ccls.navigate","args":{"direction":"U"}}]},
{"before":["<leader>","l"],"commands":[{"command":"ccls.navigate","args":{"direction":"D"}}]}
]