E2E Testing
This page contains a set of tips and tricks to help the contributor write End-to-End tests using Playwright
Your server needs to be up and going with TEST_MODE=true
env to be able to run the e2e tests locally.
TEST_MODE=true yarn dev
To run the tests locally you can run the following command:
yarn test:e2e
You can also run a specific test by providing the file name after the command, check other CLI parameters here:
yarn test:e2e engagement-dashboard.spec.ts
OR
You can also run the tests using the Playwright VS Code extension from the marketplace, check the usage reference here
Speaking specifically of e2e tests, we understand that we want to test exactly what the user sees or accesses.
The user doesn't understand HTML classes or attributes.
In this way, we concluded that the best way to find the elements is through the role
selectors https://playwright.dev/docs/selectors#role-selector
That way we go straight to the point, and we guarantee that not only the tests have access to the elements, but that users will most likely have the same type of access.
of course, sometimes in special cases, it will not be possible to identify some elements without the help of a context, for that we suggest the use of data-qa-*
attributes
To capture an element inside a page/element/component is recommended to use data-attributes
besides the normal DOM elements or capture by element position.
The recommended data-attributes
are:
data-qa
: High order testing component/page/element
data-qa-id
: Unique identification for an element
data-qa-type
: The type of an element, could be also a state
playwright.config.ts
development configuration:
- Capture the users and admin session from your local code, you need to run the code for first time without the
globalSetup
commented to generate the JSON, after the first run you can comment again to make the execution of the tests faster. - Always open the network to make it easier to debug (
'--auto-open-devtools-for-tabs'
) - Don't forget to dismiss the changes before committing
import type { PlaywrightTestConfig } from '@playwright/test';
import * as constants from './tests/e2e/config/constants';
export default {
// globalSetup: require.resolve('./tests/e2e/config/global-setup.ts'),
use: {
headless: true,
ignoreHTTPSErrors: true,
trace: 'retain-on-failure',
baseURL: constants.BASE_URL,
screenshot: process.env.CI ? 'off' : 'only-on-failure',
channel: 'chrome',
launchOptions: {
// force GPU hardware acceleration
// (even in headless mode)
args: ['--use-gl=egl', '--auto-open-devtools-for-tabs', '--use-fake-ui-for-media-stream'],
},
permissions: ['microphone'],
},
outputDir: 'tests/e2e/.playwright',
reporter: process.env.CI ? 'github' : 'list',
testDir: 'tests/e2e',
workers: process.env.CI ? 2 : undefined,
timeout: process.env.CI ? 2000_000 : 600_000,
retries: process.env.CI ? 2 : undefined,
globalTimeout: 2000_000,
maxFailures: process.env.CI ? 5 : undefined,
} as PlaywrightTestConfig;
-
If some tests fail in the GitHub CI you can go to Summary:
-
Select and download the Artifacts test that failed:
-
Extract the downloaded ZIP file
-
Inside the extracted file you will find all the failed tests with a folder called trace.zip
-
Go to a Terminal and run the command
npx playwright show-trace
or open the website Trace Playwright -
A Playwright Window will open and you can drag the trace.zip folder inside the window
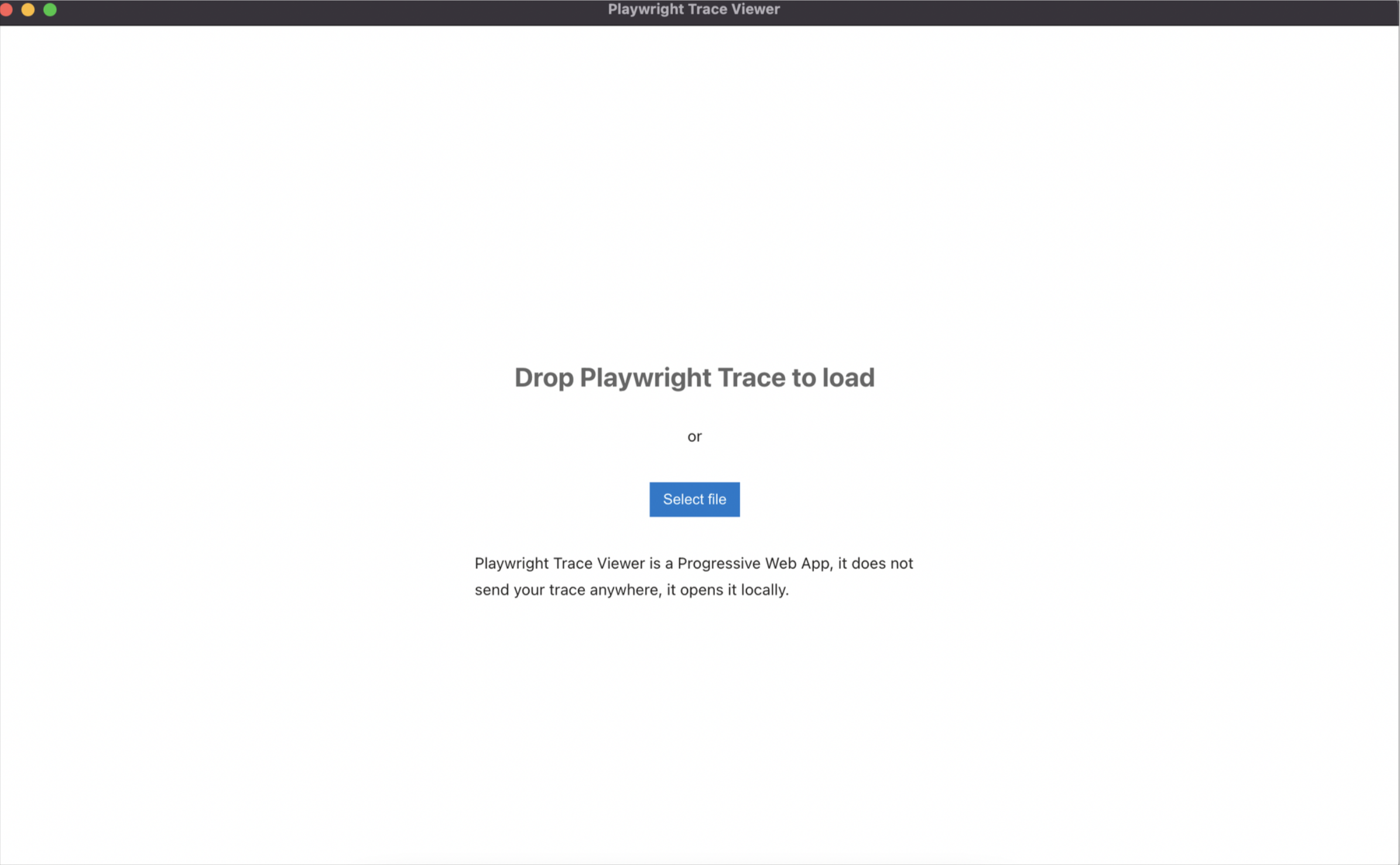
- Then you can debug and see all details about the failed tests
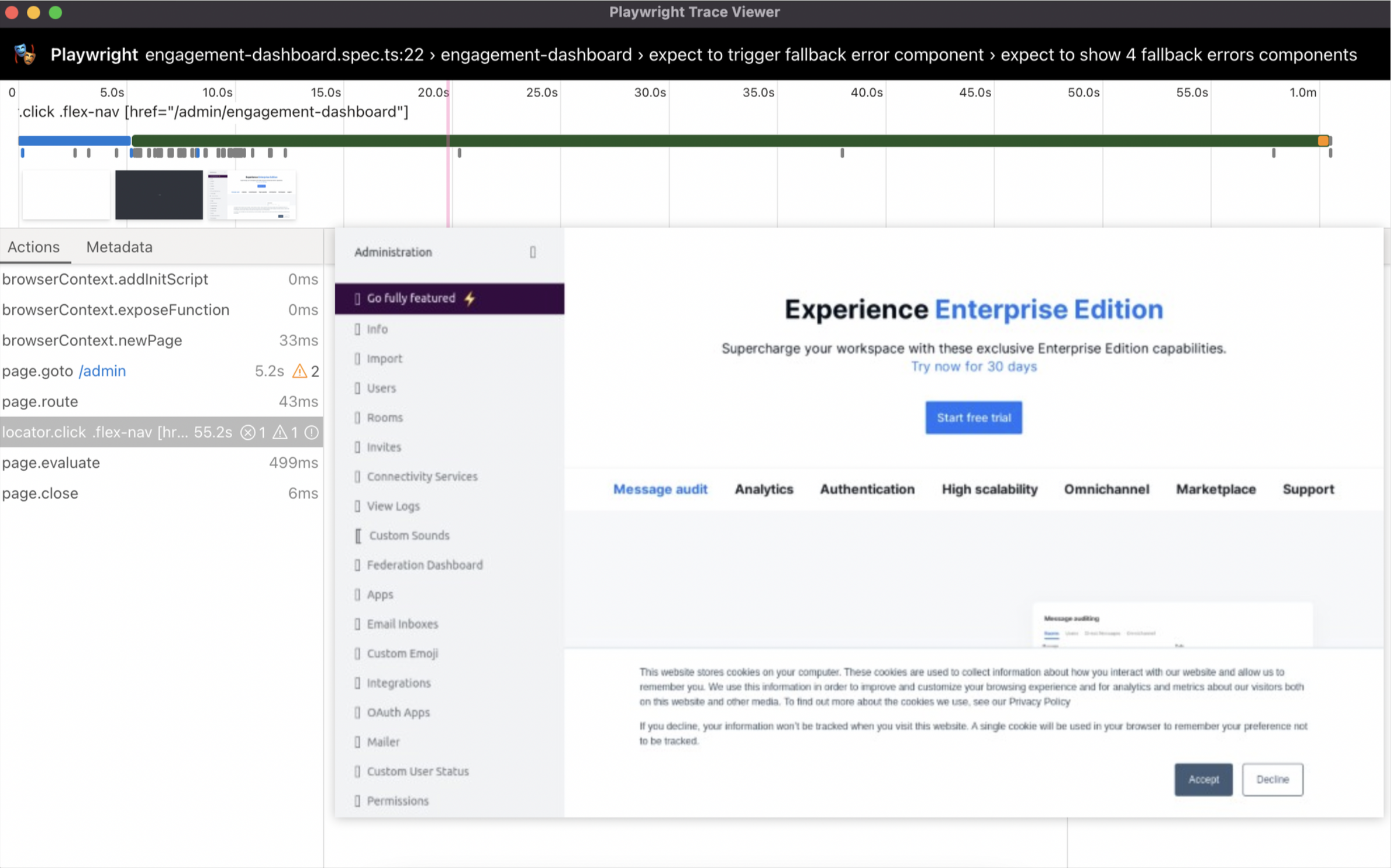
To develop some tests just for enterprise edition features, it is necessary to add the license manually and run the desired Enterprise Edition Features tests locally.
To succeed at the GitHub CI you can add a conditional skip
verifying if it has an enterprise edition license, using the constant IS_EE
, if it has not had an Enterprise Edition license the tests below this condition will be skipped
test.skip(!IS_EE, 'Enterprise Only');
The Enterprise Edition license is applied at the test-ee RUN
OR
To run locally you can run the following command:
IS_EE=true yarn test:e2e