Commons
- Introducing
ImagePickerSheetView
! This is a SheetView that can be used to pick images from local storage, while also offering placeholder options for camera and picking. Android doesn't have a nice built-in option for having the user choose between a camera or local storage, so hopefully this will be a good utility for the community.
- Check out the sample for some example usage, including intents to use for camera and picker tiles.
ImagePickerSheetView sheetView = new ImagePickerSheetView.Builder(this)
.setMaxItems(30)
.setShowCameraOption(createCameraIntent() != null)
.setShowPickerOption(createPickIntent() != null)
.setImageProvider(new ImagePickerSheetView.ImageProvider() {
@Override
public void onProvideImage(ImageView imageView, Uri imageUri, int size) {
Glide.with(ImagePickerActivity.this)
.load(imageUri)
.centerCrop()
.crossFade()
.into(imageView);
}
})
.setOnTileSelectedListener(new ImagePickerSheetView.OnTileSelectedListener() {
@Override
public void onTileSelected(ImagePickerSheetView.ImagePickerTile selectedTile) {
bottomSheetLayout.dismissSheet();
if (selectedTile.isCameraTile()) {
dispatchTakePictureIntent();
} else if (selectedTile.isPickerTile()) {
startActivityForResult(createPickIntent(), REQUEST_LOAD_IMAGE);
} else if (selectedTile.isImageTile()) {
showSelectedImage(selectedTile.getImageUri());
} else {
genericError();
}
}
})
.setTitle("Choose an image...")
.create();
bottomSheetLayout.showWithSheetView(sheetView);
- Fix: GridView columns not appearing sometimes on API < 17
- Improvement: Sheets on tablets will now default to a reasonable width, and will no longer default/force
MATCH_PARENT
. You can still use MATCH_PARENT
by setting your own LayoutParams
on the sheet view before passing it in.
- Improvement: Sheets on both phones and tablets will default to
WRAP_CONTENT
. You can still use MATCH_PARENT
by setting your own LayoutParams
on the sheet view before passing it in.
- Improvement: Icons in the intent picker sheet are lazily loaded in the background, which should substantially reduce delay when opening.
- Breaking API change:
OnIntentPickedListener#onIntentPicked
will now return the full ActivityInfo
rather than the intent. This is to provide more flexibility and allow usage of its new tag
field for storing other information.
- To recreate the concrete intent, you can call the new
getConcreteIntent(Intent)
method.
public Intent getConcreteIntent(Intent intent) {
Intent concreteIntent = new Intent(intent);
concreteIntent.setComponent(componentName);
return concreteIntent;
}
BottomSheetLayout
- New: Added methods for setting custom peek translations (@davideas)
- New: Added two helper functions:
predictedDefaultWidth
and isTablet
. These are for retrieving what BottomSheetLayout
thinks its width and whether or not it is on a tablet.
- Fix: Sheets not staying anchored to the bottom of the screen in some places
- Fix: InterceptContentTouch flag not being handled correctly, which would sometimes cause problems with intercepting touches on the sheet view
- Fix: Prevent the layout from rendering the sheet undismissable in race conditions (#48)
Sample
- We've included an ImagePicker demo, complete with image loading and intent handling.
Enjoy!
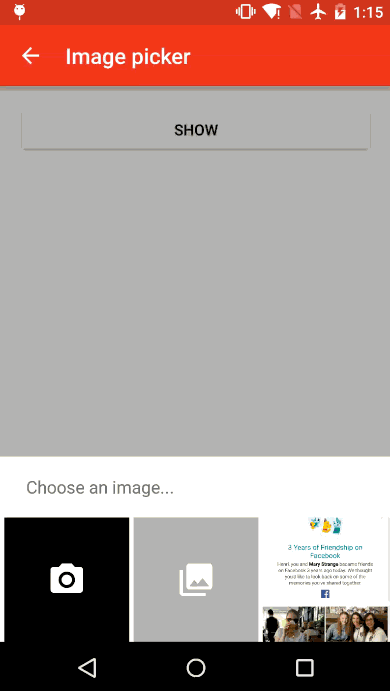