You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
functioncurry(fn){returnfunctioncurried(...args){// 1. if enough args, call func// 2. if not enough, bind the args and wait for new oneif(args.length>=fn.length){// 'this' is pointing to Window, apply is used to call the function fn//console.log(this)returnfn.apply(this,args)}else{// 'this' is pointing to Window//console.log(this)returncurried.bind(this, ...args)}}}
Using arrow function, this is no need to apply or bind, since 'this' in arrow function inherited from the parent scope
Currying is a useful technique used in JavaScript applications.
Please implement a curry() function, which accepts a function and return a curried one.
Here is an example
Regular function
Using arrow function, this is no need to apply or bind, since 'this' in arrow function inherited from the parent scope
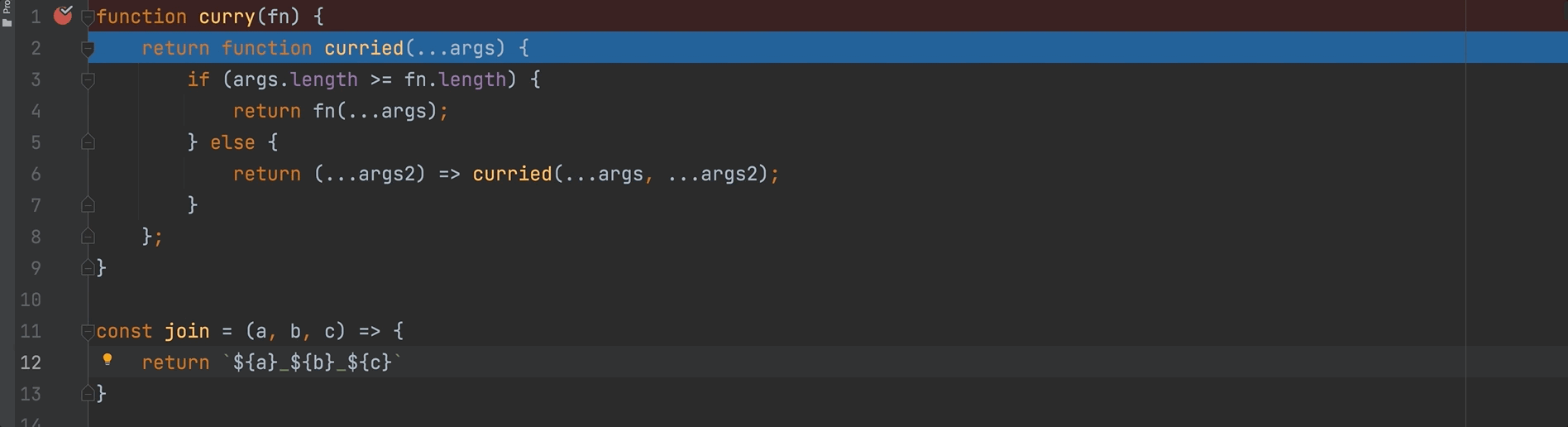
The text was updated successfully, but these errors were encountered: