We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Who faced with having problem for maxlines can use this:
Just Modify TypewriterAnimatedText
TypewriterAnimatedText
to
import 'package:flutter/material.dart'; import 'animated_text.dart'; /// Animated Text that displays a [Text] element as if it is being typed one /// character at a time. Similar to [TyperAnimatedText], but shows a cursor. /// /// 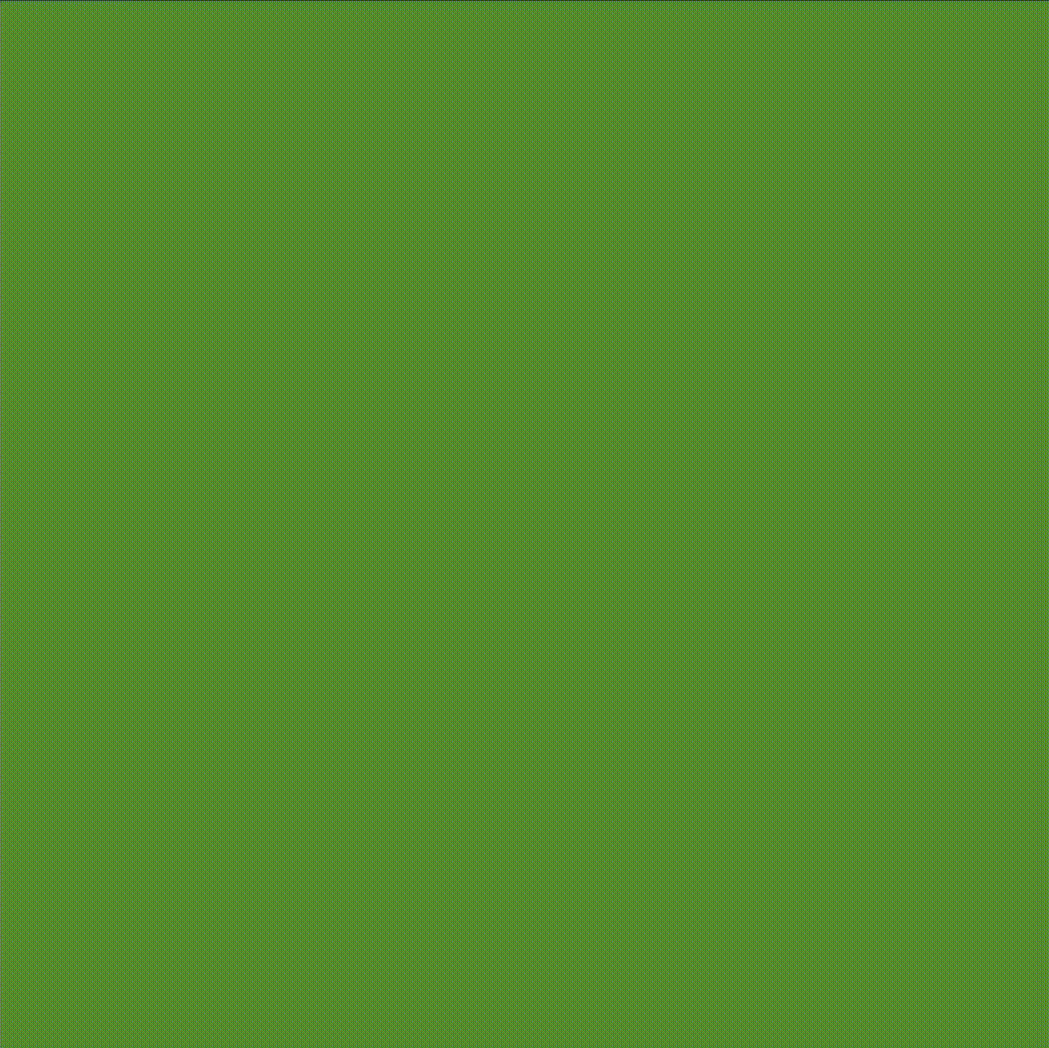 class TypewriterAnimatedText extends AnimatedText { // The text length is padded to cause extra cursor blinking after typing. static const extraLengthForBlinks = 8; /// The [Duration] of the delay between the apparition of each characters /// /// By default it is set to 30 milliseconds. final Duration speed; /// The [Curve] of the rate of change of animation over time. /// /// By default it is set to Curves.linear. final Curve curve; /// Cursor text. Defaults to underscore. final String cursor; final int? maxLines; TypewriterAnimatedText( String text, { TextAlign textAlign = TextAlign.start, TextStyle? textStyle, this.speed = const Duration(milliseconds: 30), this.curve = Curves.linear, this.maxLines, this.cursor = '_', }) : super( text: text, textAlign: textAlign, textStyle: textStyle, duration: speed * (text.characters.length + extraLengthForBlinks), ); late Animation<double> _typewriterText; @override Duration get remaining => speed * (textCharacters.length + extraLengthForBlinks - _typewriterText.value); @override void initAnimation(AnimationController controller) { _typewriterText = CurveTween( curve: curve, ).animate(controller); } @override Widget completeText(BuildContext context) => RichText( text: TextSpan( children: [ TextSpan(text: text), TextSpan( text: cursor, style: const TextStyle(color: Colors.transparent), ) ], style: DefaultTextStyle.of(context).style.merge(textStyle), ), maxLines: maxLines, // Apply maxLines here textAlign: textAlign, ); /// Widget showing partial text @override Widget animatedBuilder(BuildContext context, Widget? child) { /// Output of CurveTween is in the range [0, 1] for majority of the curves. /// It is converted to [0, textCharacters.length + extraLengthForBlinks]. final textLen = textCharacters.length; final typewriterValue = (_typewriterText.value.clamp(0, 1) * (textCharacters.length + extraLengthForBlinks)) .round(); var showCursor = true; var visibleString = text; if (typewriterValue == 0) { visibleString = ''; showCursor = false; } else if (typewriterValue > textLen) { showCursor = (typewriterValue - textLen) % 2 == 0; } else { visibleString = textCharacters.take(typewriterValue).toString(); } return RichText( text: TextSpan( children: [ TextSpan(text: visibleString), TextSpan( text: cursor, style: showCursor ? null : const TextStyle(color: Colors.transparent), ) ], style: DefaultTextStyle.of(context).style.merge(textStyle), ), maxLines: maxLines, // Apply maxLines here textAlign: textAlign, ); } } /// Animation that displays [text] elements, as if they are being typed one /// character at a time. Similar to [TyperAnimatedTextKit], but shows a cursor. /// /// 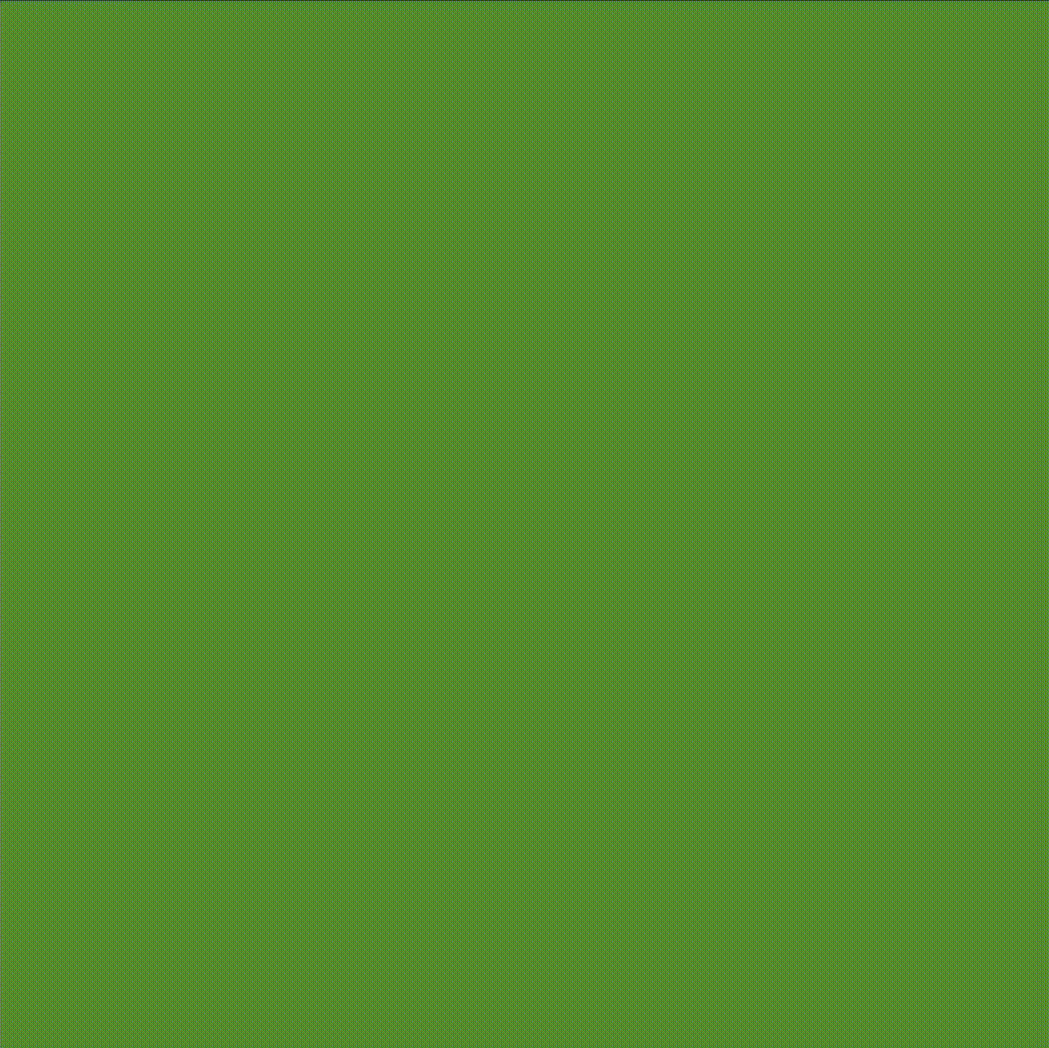 @Deprecated('Use AnimatedTextKit with TypewriterAnimatedText instead.') class TypewriterAnimatedTextKit extends AnimatedTextKit { TypewriterAnimatedTextKit({ Key? key, required List<String> text, TextAlign textAlign = TextAlign.start, required TextStyle textStyle, Duration speed = const Duration(milliseconds: 30), Duration pause = const Duration(milliseconds: 1000), bool displayFullTextOnTap = false, bool stopPauseOnTap = false, VoidCallback? onTap, void Function(int, bool)? onNext, void Function(int, bool)? onNextBeforePause, VoidCallback? onFinished, bool isRepeatingAnimation = true, bool repeatForever = true, int totalRepeatCount = 3, Curve curve = Curves.linear, }) : super( key: key, animatedTexts: _animatedTexts(text, textAlign, textStyle, speed, curve), pause: pause, displayFullTextOnTap: displayFullTextOnTap, stopPauseOnTap: stopPauseOnTap, onTap: onTap, onNext: onNext, onNextBeforePause: onNextBeforePause, onFinished: onFinished, isRepeatingAnimation: isRepeatingAnimation, repeatForever: repeatForever, totalRepeatCount: totalRepeatCount, ); static List<AnimatedText> _animatedTexts( List<String> text, TextAlign textAlign, TextStyle textStyle, Duration speed, Curve curve, ) => text .map((_) => TypewriterAnimatedText( _, textAlign: textAlign, textStyle: textStyle, speed: speed, curve: curve, )) .toList(); }
The text was updated successfully, but these errors were encountered:
No branches or pull requests
Who faced with having problem for maxlines can use this:
Just Modify
TypewriterAnimatedText
to
The text was updated successfully, but these errors were encountered: