You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
and you will see: 'My Address Value' instead of 'Updated Address Value'.
Possible Solutions
This package should not call mutators and casters to alter the values.
Every Auditable model should be able to transform itself when you create a new instance of the model passing the new attributes.
This is what I'm doing. See gist.
Basically creating a class that will create the oldAuditableModel and newAuditableModel.
And laravel will take care of mutators and casting. The setRawAttributes function does the trick here.
Actual Behaviour
Following your documentation: "The getModified() method honors all attribute mutators and/or casts defined in the Auditable model, transforming the data accordingly."
The issue is when casting to value objects. See laravel documentation.
When this method is calling "getFormattedValue" laravel will get the value from the classCastCache property. See: https://github.com/laravel/framework/blob/master/src/Illuminate/Database/Eloquent/Concerns/HasAttributes.php#L562
$modified['old'] will be equal to $modified['new'] if your attribute is using a custom cast.
Expected Behaviour
$modified['old'] should NOT be equal to $modified['new'] if the attribute is using a custom cast.
Steps to Reproduce
1- First create a cast class
2- Add the cast to the model
3- Update the model
4- In your view call this.
and you will see: 'My Address Value' instead of 'Updated Address Value'.
Possible Solutions
This package should not call mutators and casters to alter the values.
Every Auditable model should be able to transform itself when you create a new instance of the model passing the new attributes.
This is what I'm doing. See gist.
Basically creating a class that will create the oldAuditableModel and newAuditableModel.
And laravel will take care of mutators and casting. The setRawAttributes function does the trick here.
2- Add a method displayAuditField to the Auditable model to return the proper value. This method is way to sanitize the input and avoid errors.
3- Then in your view you can do this
Image:
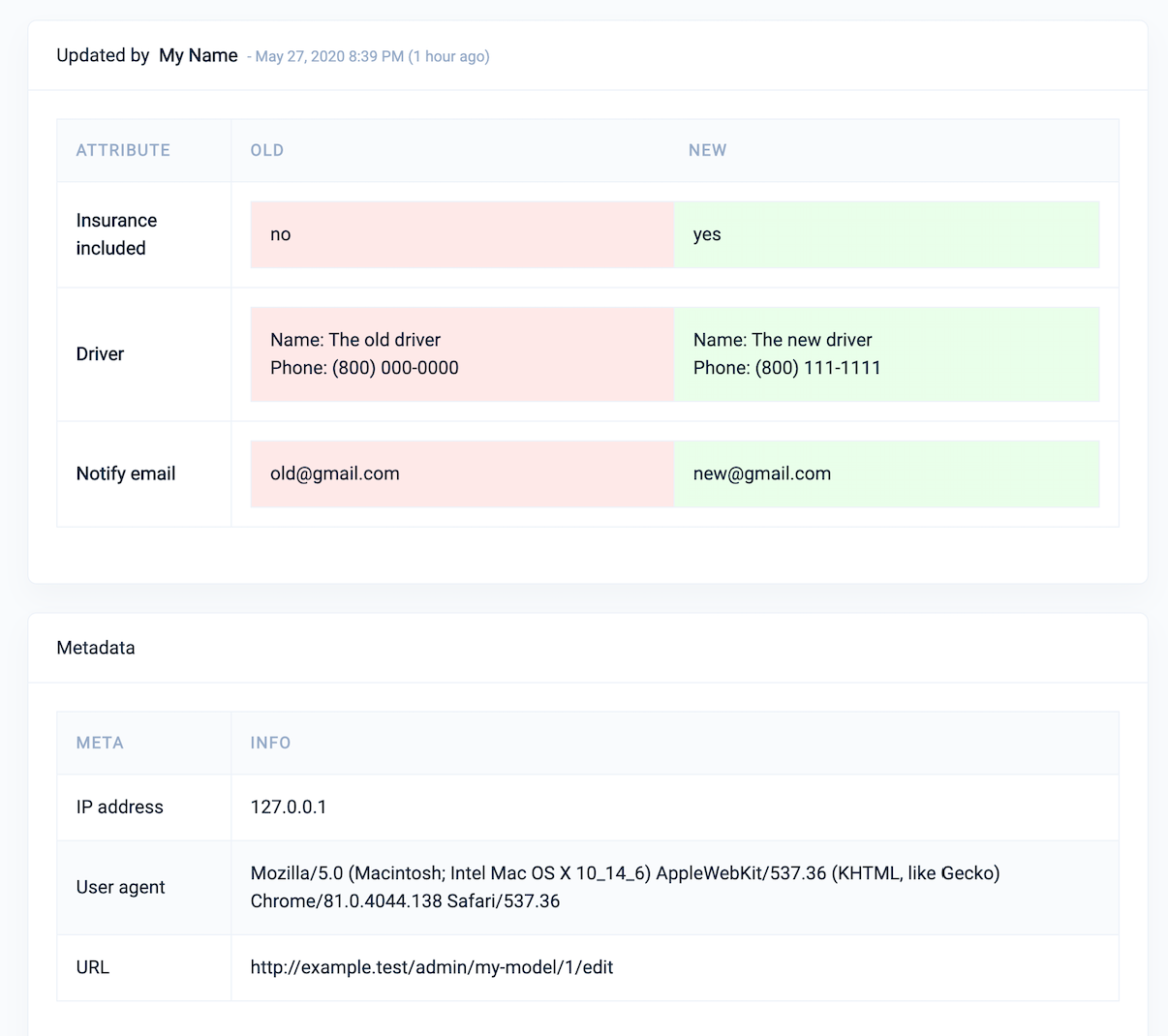
The text was updated successfully, but these errors were encountered: