-
Notifications
You must be signed in to change notification settings - Fork 52
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Circle/Rectangle Collision Detection Response Issue! #10
Comments
There is a response! Or, more specifically, the functions return The
That final If I understand correctly, you want to keep the circle from entering the square. If it's moving automatically, you could just not update the position if there is a hit.
If you wanted the circle to bounce back, you'd need to do something like:
To do this with a mouse will be a little trickier – probably using Does that answer your question? |
Thanks for replying. I know this method. The circle rubs along the edge of the square with your method, as I wanted, it's worked. But It's not an accurate method! Because when I change the speed and the circle stays far away from the square more than before. Your method means that when the circle touches to the square and then, minus Circle's Speed from the Circle's X and Y position. Yeah, it works a little bit correct just with a small speed. But When I change the speed a little bit of the circle and then it returns with the different wrong value! You can see in this video => https://www.youtube.com/watch?v=h_ti1cx5tCA&feature=youtu.be Please watch without skipping, This response is not accurate!
I used your method in Square/Square and Circle/Circle before, and then I saw that this is not an accurate way for a response. And I left this way! I do not use this method! Please visit my website => http://learngraphx.byethost12.com/opengl-17.html and there you will see Circle to circle collision detection with a response in another method. I could use it with your method in Circe/Circle too, but it's not accurate, but I used another way for Square/Square and Circle/Circle Collision Detection Response, please check out my website, and you can see, and scroll down to watch the result of Circle/Circle Collision Detection Response in my website! Below you can see, my responses methods for Square/Square and Circle/Circle:
And as you can see that I used another method for Square/Square and Circle/Circle Collision Detection for Response! And it did not take a lot more work as you said. In my response methods if I change the speed of the circle or square and still it returns a correct value with my methods in Square/Square and Circle/Circle, but with your methods returns a different wrong value each time when I change the speed. I made Square/Square, Circle/Circle collision detection with correct responses, and now I have stuck in Circle/Square Collision Detection Response. I can not figure out how to calculate for the Circle/Square Collision Detection Response. I want an accurate method for Circle/Square Collision Detection Response like mine responses! For example like this:
|
I'm going to have to take a look at your post a little more closely when I have a minute, but I think you're describing the "bullet through paper problem." If the step of your object (speed) is too far, it can miss triggering the collision. It's mentioned at the bottom of this example in the book and has been on my list of things to add to the site when I have time. For now, you could try the resources I've listed there for potential solutions. |
Not a problem! When you have time then add responses to your site! |
There is no problem with the collision detection test! It works! But there is not a collision detection response! I want that when the circle touches the square, and the circle must stay outside of the rectangle! Image =>
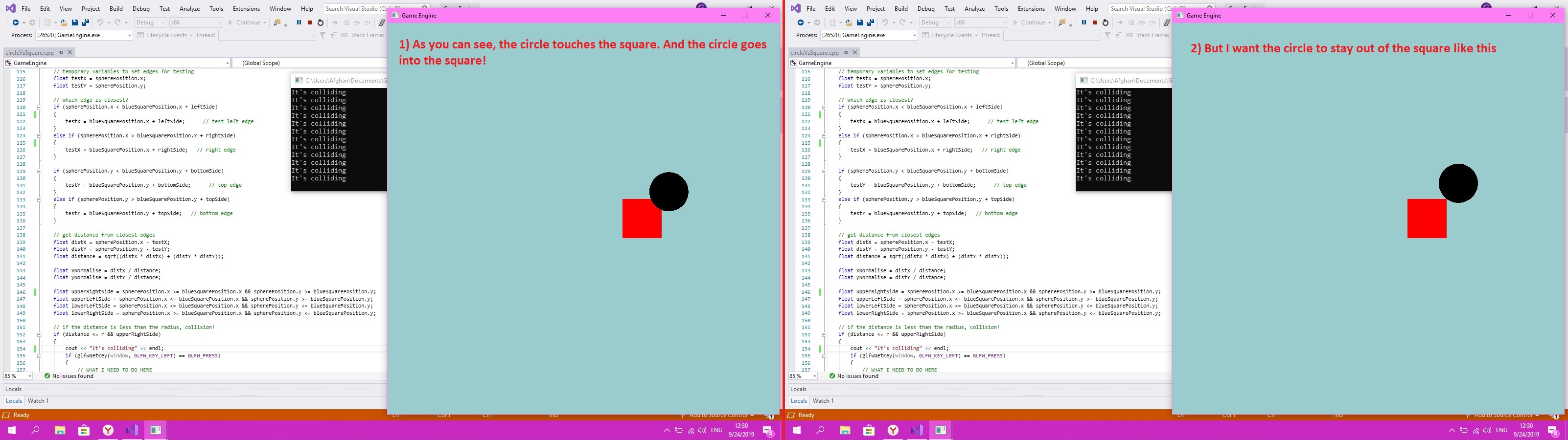
The text was updated successfully, but these errors were encountered: