New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Resizing with snap does not update drawable area #126
Comments
Seems to work for me on macOS. Maybe you need to trigger redraw after resize? |
How would I do so? I'd assumed the loop calling all the drawing methods would do so already |
Can you share the source? |
class Window {
// Skija values
private lateinit var context: DirectContext
private lateinit var renderTarget: BackendRenderTarget
private lateinit var surface: Surface
// Contains utilities and the canvas
private lateinit var ctx: RenderContext
private val window: Long
// initial values
private val width = 640
private val height = 480
// Seems to be needed?
private var fbId = 0
// Low numbers are rendered first
private val layers = PriorityQueue<Pair<Int, RenderLayer>>(compareBy { it.first })
init {
glfwInit()
glfwWindowHint(GLFW_VISIBLE, GLFW_FALSE)
glfwWindowHint(GLFW_RESIZABLE, GLFW_TRUE)
window = glfwCreateWindow(width, height, "Hello Window", NULL, NULL)
glfwMakeContextCurrent(window)
glfwSwapInterval(1)
glfwShowWindow(window)
// set up callbacks
glfwSetWindowSizeCallback(window) { w, width, height -> onResize(width, height) }
glfwSetKeyCallback(window) { w, key, scancode, action, mods -> onKey(key, scancode, action, mods) }
glfwSetMouseButtonCallback(window) { w, button, action, mods -> onClick(button, action, mods) }
}
fun start() {
GL.createCapabilities()
context = DirectContext.makeGL()
fbId = glGetInteger(0x8CA6) // GL_FRAMEBUFFER_BINDING
renderTarget = BackendRenderTarget.makeGL(
width,
height,
0,
8,
fbId,
FramebufferFormat.GR_GL_RGBA8
)
surface = Surface.makeFromBackendRenderTarget(
context,
renderTarget,
SurfaceOrigin.BOTTOM_LEFT,
SurfaceColorFormat.RGBA_8888,
ColorSpace.getSRGB()
)
ctx = RenderContext(surface.canvas)
// Render loop
while (!glfwWindowShouldClose(window)) {
surface.canvas.clear(0xFF000000.toInt() + ctx.theme.BACKGROUND)
layers.forEach {
it.second.render(ctx)
}
context.flush()
glfwSwapBuffers(window)
glfwPollEvents()
}
surface.close()
renderTarget.close()
context.close()
glfwFreeCallbacks(window);
glfwDestroyWindow(window);
glfwTerminate();
glfwSetErrorCallback(null)?.free();
}
fun addLayer(priority: Int, layer: RenderLayer) {
layers.add(Pair(priority, layer))
}
private fun onResize(width: Int, height: Int) {
// Create new backend
val newBackend = BackendRenderTarget.makeGL(
width,
height,
0,
8,
fbId,
FramebufferFormat.GR_GL_RGBA8
)
val newSurface = Surface.makeFromBackendRenderTarget(
context,
renderTarget,
SurfaceOrigin.BOTTOM_LEFT,
SurfaceColorFormat.RGBA_8888,
ColorSpace.getSRGB()
)
// Swap (should reduce issues from using closed backend, assuming this runs on a separate thread)
val oldSurface = surface
val oldBackend = renderTarget
val oldCtx = ctx
renderTarget = newBackend
surface = newSurface
ctx = RenderContext(surface.canvas)
// Close old backend
oldSurface.close()
oldBackend.close()
oldCtx.textRenderer.close()
println("onResize $width $height")
ResizeEvent.EVENT.invoker().invoke(width, height)
}
private fun onKey(key: Int, scancode: Int, action: Int, mods: Int) {
println("onKey $key $scancode $action $mods")
KeyEvent.EVENT.invoker().invoke(key, scancode, action, mods)
}
private fun onClick(button: Int, action: Int, mods: Int) {
println("onClick $button $action $mods")
// TODO: Event
}
} it clearly logs |
I've been able to confirm this affects multiple devices and multiple OSes. |
Have you tried adding draw to the end of resize handler? That’s what I am doing in LWJGL example, and I don’t see any artefacts (apart from resize being late for ~1 frame, but that’s expected from GLFW): skija/examples/lwjgl/src/Main.java Lines 154 to 156 in 5fb057b
What OSes have you tested this on? Also, I don’t think I understad your issue completely. What exactly are you doing (step by step), and what are you seeing? What do you mean by “snap to a side”? When you say “it requires an additional resize to actually trigger the surface reload” do you mean it renders at the wrong size permanently or just for 1 frame? |
I recorded my screen to show how I can reproduce the issue 2021-08-15_21-05-16.mp4 |
I checked with our LWJGL example (which is based on GLFW) and Ubuntu 20.04, seems to work fine. Not sure what’s going on in your case. Are you sure you get correct width/height in onResize callback? Have you tried moving oldSurface.close()/oldBackend.close() before creating new ones? |
Can you check too, btw? Clone this and run |
looks like it fails to compile when running that command with java 11 set, and with java 16:
Note that in both cases nothing visually appeared. |
Yes
Yes, that still has the same issue. |
Remove assert from updateDimensions? |
I made a sample app using lwjgl as backend that just draws some text in the corner:
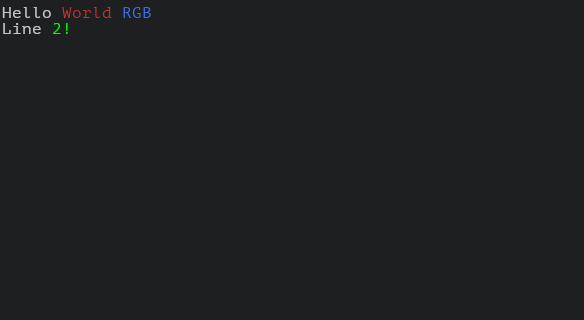
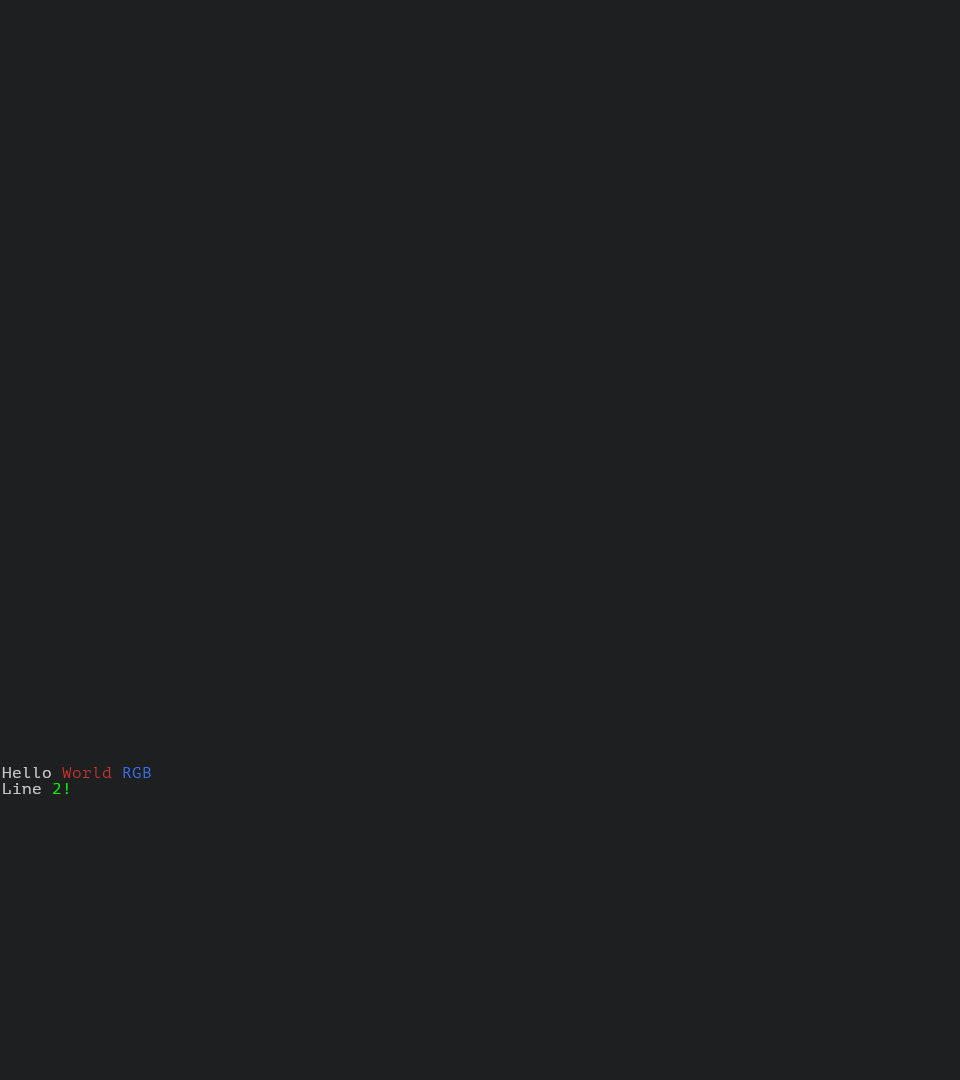
This works fine when manually resizing to any size. However, once I start making it snap to a side, it does not update the surface correctly*:
It does tell me it resized to fullscreen in the handler callback though.
The code handling resizing is as follows:
*it seems it requires an additional resize to actually trigger the surface reload.
The text was updated successfully, but these errors were encountered: